• Registers are for sequential circuits necessary, so will be inferred by the compiler.
• This should not happen for combinational circuits.
• Also for a combinational circuit, the complete truth-table should be clearly specified in the code.
Rules to be followed while using sequential code for designing combinational circuits
• Rule 1: Make sure that all input signals used (read) in the PROCESS appear in its sensitivity list.
• Rule 2: Make sure that all combinations of the input/output signals are included in the code; that is, make sure that, by looking at the code, the circuit’s complete truth-table can be obtained (indeed, this is true for both sequential as well as concurrent code).
• Failing to comply with rule 1 will generally cause the compiler to simply issue a warning saying that a given input signal was not included in the sensitivity list, and then proceed as if the signal were included. Even though no damage is caused to the design in this case, it is a good design practice to always take rule 1 into consideration.
• With respect to rule 2, however, the consequences can be more serious because incomplete specifications of the output signals might cause the synthesizer to infer latches in order to hold their previous values.
Bad Combinational Design
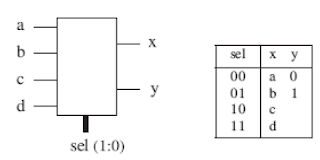
x should behave as a multiplexer; that is, should be equal to the input selected by sel;
y, on the other hand, should be equal to ‘0’ when sel = ‘‘00’’, or ‘1’ if sel = ‘‘01’’
LIBRARY ieee;
USE ieee.std_logic_1164.all;
ENTITY example IS
PORT (a, b, c, d: IN STD_LOGIC; sel: IN INTEGER RANGE 0 TO 3;
x,y: OUT STD_LOGIC);
END example;
ARCHITECTURE example OF example IS
BEGIN
PROCESS (a, b, c, d, sel)
BEGIN
IF (sel=0) THEN
x<=a;
y<=‘0’;
ELSIF (sel=1) THEN
x<=b;
y<=‘1’;
ELSIF (sel=2) THEN
x<=c;
ELSE
x<=d;
END IF;
END PROCESS;
END example;
Output
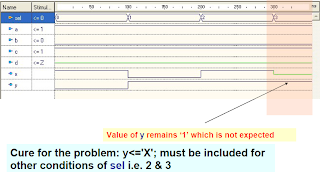
Some templates for use!
process (Inputs) -- All inputs in sensitivity list
begin
... -- Outputs assigned for all input conditions
... -- No feedback
end process; -- Gives pure combinational logic
process (Inputs) -- All inputs in sensitivity list
begin
if Enable = '1' then
... -- Latched actions
end if;
end process; -- Gives transparent latches + logic
process (Clock)
-- Clock only in sensitivity list
begin
if Rising_edge(Clock) then -- Test clock edge only
...
-- Synchronous actions
end if;
end process;
-- Gives flipflops + logic
process (Clock, Reset) -- Clock and reset only in -- sensitivity list
begin
if Reset = '0' then -- Test active level of -- asynchronous reset
... -- Asynchronous actions
elsif Rising_edge(Clock) then -- Test clock edge -- only
... -- Synchronous actions
end if;
end process; -- Gives flipflops + logic
process -- No sensitivity list
begin
wait until Rising_edge(Clock);
... -- Synchronous actions
end process; -- Gives flipflops + logic
information shared by www.irvs.info