• Like IF, WAIT, and CASE, LOOP is intended exclusively for sequential code, so it too can only be used inside a PROCESS, FUNCTION, or PROCEDURE.
• There are several ways of using LOOP.
• A loop is an infinite loop (and thus an error) unless it contains an exit or wait statement.
Syntax
[label:] LOOP
(SequentialStatements)
END LOOP [label];
FOR / LOOP: The loop is repeated a fixed number of times
[label:] FOR identifier IN range LOOP
(sequential statements)
END LOOP [label];
WHILE / LOOP: The loop is repeated until a condition no longer holds
[label:] WHILE condition LOOP
(sequential statements)
END LOOP [label];
EXIT: Used for ending the loop
[label:] EXIT [label] [WHEN condition];
NEXT: Used for skipping loop steps
[label:] NEXT [loop_label] [WHEN condition];
WAIT : Continue looping until..
Example of FOR / LOOP:
FOR i IN 0 TO 5 LOOP
x(i) <= enable AND w(i+2);
y(0, i) <= w(i);
END LOOP;
NOTE: The loop will be repeated unconditionally until i reaches 5
(that is, six times)
• One important remark regarding FOR /LOOP is that both limits of the range must
be static.
• Thus a declaration of the type "FOR i IN 0 TO choice LOOP",where choice is an input (non-static) parameter, is generally not synthesize.
Example of WHILE / LOOP:
WHILE (i < 10) LOOP
WAIT UNTIL clk'EVENT AND clk='1';
(other statements)
END LOOP;
Example of WAIT in LOOP :
LOOP
WAIT UNTIL Clock = '1';
EXIT WHEN Reset = '1';
Div2 <= NOT Div2;
END LOOP;
Example of EXIT :
FOR i IN data'RANGE LOOP
CASE data(i) IS
WHEN '0' => count:=count+1;
WHEN OTHERS => EXIT;
END CASE;
END LOOP;
NOTE: EXIT implies not an escape from the current iteration of the loop, but rather a definite exit (that is, even if i is still within the data range, the LOOP statement will be considered as concluded).
In this case, the loop will end as soon as a value different from ‘0’ is found in the data vector.
Example with NEXT:
FOR i IN 0 TO 15 LOOP
NEXT WHEN i=skip; -- jumps to next iteration
(...)
END LOOP;
NOTE: NEXT causes LOOP to skip one iteration
when i = skip
Synthesis
• Not generally synthesizable. Some tools do allow loops containing wait statements to describe implicit finite state machines, but this is not a recommended practice.
Example : 8-bit unsigned Carry Ripple Adder
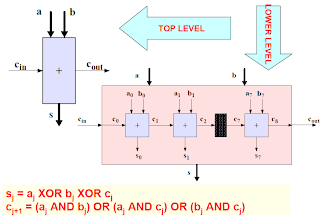
LIBRARY ieee;
USE ieee.std_logic_1164.all;
ENTITY adder IS
GENERIC (length : INTEGER := 8);
PORT ( a, b: IN STD_LOGIC_VECTOR (length-1 DOWNTO 0);
cin: IN STD_LOGIC; s: OUT STD_LOGIC_VECTOR (length-1 DOWNTO 0);
cout: OUT STD_LOGIC);
END adder;
ARCHITECTURE adder OF adder IS
BEGIN
PROCESS (a, b, cin)
VARIABLE carry : STD_LOGIC_VECTOR (length DOWNTO 0);
BEGIN
carry(0) := cin;
FOR i IN 0 TO length-1 LOOP
s(i) <= a(i) XOR b(i) XOR carry(i);
carry(i+1) := (a(i) AND b(i)) OR (a(i) AND
carry(i)) OR (b(i) AND carry(i));
END LOOP;
cout <= carry(length);
END PROCESS;
END adder;
Output
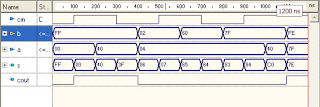
Example: Leading Zeros
The circuit should count the number of leading zeros in a binary vector, starting from the left end.
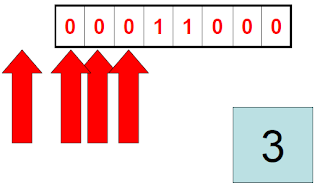
LIBRARY ieee;
USE ieee.std_logic_1164.all;
ENTITY LeadingZeros IS
PORT ( data: IN STD_LOGIC_VECTOR (7 DOWNTO 0);
zeros: OUT INTEGER RANGE 0 TO 8);
END LeadingZeros;
ARCHITECTURE behavior OF LeadingZeros IS
BEGIN
PROCESS (data)
VARIABLE count: INTEGER RANGE 0 TO 8;
BEGIN
count := 0;
FOR i IN data'RANGE LOOP
CASE data(i) IS
WHEN '0' => count := count + 1;
WHEN OTHERS => EXIT;
END CASE;
END LOOP;
zeros <= count;
END PROCESS;
END behavior;
Output
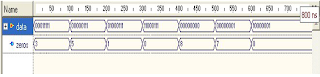
information shared by www.irvs.info